Greetings, everyone! My name is Rishabh Deshpande. Today, we’re going to dive into the world of Python programming in the most straightforward and accessible manner possible. Let’s begin!
Indeed, the journey of every programmer often begins with a simple yet significant piece of code: the “Hello, World!” program.
print("Hello world")
Hello world
In the code provided, you see the print
function. We’ll delve into functions later in our learning journey.
table of contents
Let’s learn about VARIABLES in PYTHON PROGRAMMING
Absolutely! Variables are fundamental in programming. They are like containers that hold data which can change over time. In Python, you can declare a variable and assign a value to it using the following syntax:
Variable_1 = value
name = "Rishabh"
Age = 26
CGPA = 9.02
Here , Age is a variable that holds an integer value (26), and Name is a variable that holds a string value (“Rishabh”). CGPA hold the Floating value.
Variables in Python can hold different types of data, such as integers, floating-point numbers, strings, lists, dictionaries, and more. Python is dynamically typed, meaning you don’t need to explicitly declare the type of a variable; Python infers it based on the value assigned to it.
Let’s create a Python script in VS Code and write some examples based on what we’ve learned about variables.
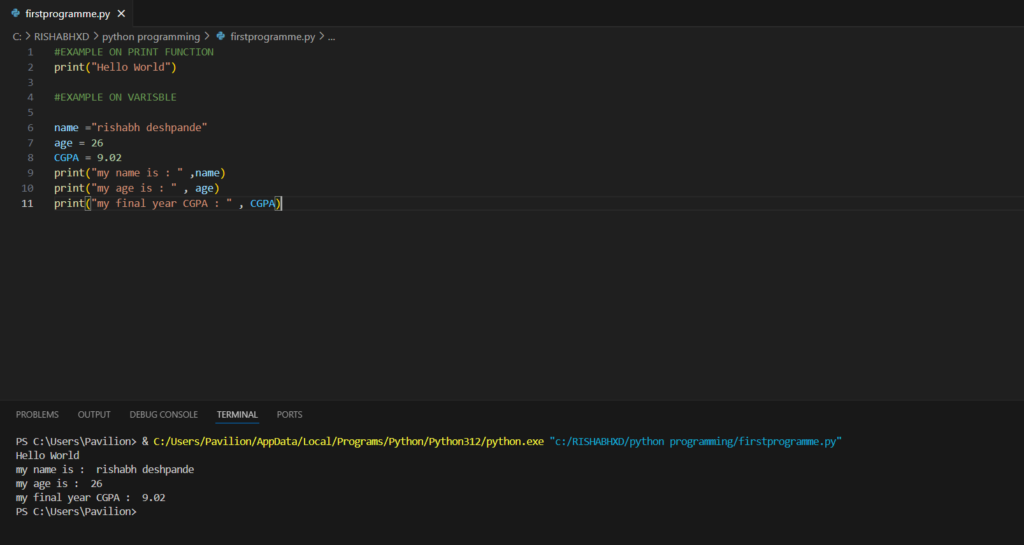
Let’s learn about EXPRESSION EXECUTION in PYTHON PROGRAMMING
- String and number values can operate together with *
#INPUT
A,B=2,3
TXT="@"
print(A*TXT*B)
#OUTPUT
@@@@@@
2. String and string can operate with +
#INPUT
A,B="2",3
TXT="@"
print((A+TXT)*B)
#OUTPUT
2@2@2@
3. Numeric value can operate with all operators.
#INPUT
A,B=5,2
C=10
print(A+B*C)
#OUTPUT
25
4. Arithmetic expressions with integer and float will result in float value.
#INPUT
A,B=5,2.0
C=A*B
print(C)
#OUTPUT
10.0
5. The result of the division operator with two integers will be a float value.
#INPUT
A,B=4,2
C=A/B
print(C)
#OUTPUT
2.0
6. Integer division // with float and integer will give an integer but displayed as a float value.
#INPUT
A,B=1.5,3
C=1.5//3
print(C)
#OUTPUT
0.0
NOTE :- Integer division round of the closest small integer value but it shows as a float value.
7. Floor gives the closest integer, which is lesser than or equal to the float value. { Result of (A//B) is same as floor (A/B) }
#INPUT
A,B=12,5
C= A//B
print(C)
#OUTPUT
2
NOTE :- 12/5 ans is 2.4 but its round of to the lesser closest value. that is 2.
8. Remainder is negative when denominator is negative.
Let’s learn about CONDITIONAL STATEMENTS in PYTHON PROGRAMMING
#CONDITIONAL STATEMENT
if-elif-else (Syntax) ----> 4 spaces
if(condition):
Statement 1
elif(condition):
Statement 2
else:
statemnt
#EXAMPLE ON CONDITIONAL STATEMENT
Let’s learn about STRINGS in PYTHON PROGRAMMING
In Python, a string is a sequence of characters enclosed within either single quotes (”), double quotes (“”) or triple quotes (”’ or “””). Strings are immutable, meaning once created, their values cannot be changed.
My_string="Hello Rishabh"
Strings can contain letters, numbers, symbols, and even special characters like newline (/n) or tab (/t).
Let’s learn about INDEXING in PYTHON PROGRAMMING
Indexing in Python refers to accessing individual elements of a sequence like strings, lists, or tuples. Each element in a sequence has an index associated with it, which denotes its position within the sequence. Indexing in Python starts from 0.
RISHABH
0123456
#Lets take some examples on indexing
my_string="thank you for visiting my blog"
print(my_string[4])
#OUTPUT
k
#note: space also count
You can also use negative indices to access elements from the end of the sequence. In this case, indexing starts from -1:
string="Hello"
print(string[-1])
#OUTPUT
o
Let’s learn about SLICING in PYTHON PROGRAMMING
Slicing in Python is a way to extract a sub-sequence (sub-string, sub-list, or sub-tuple) from a sequence (string, list, or tuple) by specifying a range of indices.
str[starting_idx:ending_idx]
#Ending index is not included.
#Examples
Eg="welcome to my blog"
print(Eg[2:8])
#output
lcome t
#note:- space also included.
Certainly! Negative indexing in slicing allows you to access elements from the end of a sequence. Here are some examples:
str="Apple"
print(str[-3:-1])
#output
pl
Let’s learn about LISTS in PYTHON PROGRAMMING
A list is a collection of items that are ordered and mutable. Lists are one of the most versatile data structures and are commonly used to store multiple items of similar or different types.
- CREATION :- Lists are created by enclosing a comma-separated sequence of items within square brackets
[]
.
list=[1,2,3,4,5,6,7,8,9]
- MUTABLE :- Unlike strings, lists are mutable, meaning you can modify their elements after they have been created.
list[5]=11
print(list)
#OUTPUT
[1,2,3,4,5,11,7,8,9]
#NEXT EXAMPLES
STUDENT=[Rohit,99.99,nagpur,26]
STUDENT[0]=Rishabh
print(STUDENT)
#OUTPUT
[Rishabh,99.99,nagpur,26]
- Heterogeneous :- Lists can contain elements of different data types.
list=[1,hello,3.14,True]
- Ordered :- Lists maintain the order of elements as they are inserted.
- Nested Lists :- Lists can contain other lists as elements, allowing for the creation of nested data structures.
nested_list=[[1,2,3],[4,5,6],[7,8,9]]
- Indexing and Slicing :- Lists support indexing and slicing operations similar to strings.
list=[1,2,3,4,5,6,7,8,9]
print(list[0])
print(list[0:3])
#OUTPUT
1
[1,2,3]
- Append and Extend :- You can add elements to a list using the append ( ) method to add a single element or extend( ) method to add multiple elements from another list.
list=[2,3,4]
list.append(9)
print(list)
#OUTPUT
[2,3,4,9]
-------x-------
list=[2,3,4]
list.extend([7,8,9])
print(list)
#OUTPUT
[2,3,4,7,8,9]
Lists are incredibly versatile and are used extensively in Python for various purposes, such as storing data, iterating over elements, and implementing algorithms.
Let’s learn about TUPLES in PYTHON PROGRAMMING
a tuple is a collection of items that are ordered and immutable. Tuples are similar to lists, but they cannot be modified once created, making them suitable for storing fixed collections of data.
- Creation :- Tuples are created by enclosing a comma-separated sequence of items within parentheses ( ).
tuples=(1,2,3,4,5,6,7,8,9)
- Immutable :- Unlike lists, tuples are immutable, meaning you cannot change, add, or remove elements after the tuple has been created.
- Ordered :- Tuples maintain the order of elements as they are inserted.
- Heterogeneous :- Tuples can contain elements of different data types.
tuples=(1,"hello",3.14,true)
- Nested Tuples :- Tuples can contain other tuples as elements, allowing for the creation of nested data structures.
nested_tuples=((1,2,3),(4,5,6),(7,8,9))
- Length :- You can find the number of elements in a tuple using the len( ) function.
tuples=(1,2,3,4,5,6,7,8,9)
print(len(list))
#OUTPUT
9
- Indexing and Slicing :- Tuples support indexing and slicing operations similar to lists.
tuples=(1,2,3,4,5,6,7,8,9)
print(tuples[1])
print(slicing[1:2])
#OUTPUT
2
(2,3)
Let’s learn about DICTIONARY in PYTHON PROGRAMMING
A dictionary is a collection of key-value pairs. Dictionaries are unordered, mutable, and can contain items of different data types. Each key in a dictionary must be unique and immutable (such as strings, numbers, or tuples), while the corresponding value can be of any data type and can be mutable or immutable.
- Creation :- Dictionaries are created by enclosing a comma-separated sequence of key-value pairs within curly braces { }.
Dict={
"name" : "Rishabh",
"age" : 26,
"cgpa" : 9.02,
"tuples" : (1,2,3,4)
"list" : [1,2,3]
}
- Accessing Values :- You can access the value associated with a key by using square brackets [ ] and specifying the key & key must be unique.
Dict={
"name" : "Rishabh",
"age" : 26,
"cgpa" : 9.02,
"tuples" : (1,2,3,4)
"list" : [1,2,3]
}
print(Dict[name])
print(Dict[tuples])
#OUTPUT
Rishabh
(1,2,3,4)
- Mutable :- Dictionaries are mutable, meaning you can modify, add, or remove key-value pairs after the dictionary has been created.
my_dict = {"name": "John", "age": 26, "city": "New York"}
my_dict["age"]=36
my_dict["gender"] = "Male"
del my_dict["city"]
#OUTPUT
{'name': 'John', 'age': 36, 'gender': 'Male'}
- Keys and Values :- You can access the keys and values of a dictionary using the Keys ( ) and Values ( ) methods, respectively.
my_dict = {"name": "John", "age": 26, "city": "New York"}
print(my_dict.keys())
print(my_dict.values())
#OUTPUT
dict_keys(['name', 'age', 'city'])
dict_values(['John', 26, 'New York'])
- Items :- You can access key-value pairs as tuples using the item( ) method.
my_dict = {"name": "John", "age": 26, "city": "New York"}
print(my_dict.items())
#OUTPUT
dict_items([('name', 'John'), ('age', 26), ('city', 'New York')])
- Membership :- You can check if a key exists in a dictionary using the in operator.
my_dict = {"name": "John", "age": 26, "city": "New York"}
print("age" in my_dict)
print("city" in my_dict)
#OUTPUT
True
True
- Length :- You can find the number of key-value pairs in a dictionary using the len( ) function.
my_dict = {"name": "John", "age": 26, "city": "New York"}
print(len(my_dict))
#OUTPUT
3
- Nested Dictionary :- Dictionary within the dictionary.
nested_dict={
"person1" :{
"name":"Rishabh",
"age":26,
"city":"nagpur"
},
"person2": {
"name": "Rohit",
"age": 25,
"city": "nagpur"
}
}
print(nested_dict)
#OUTPUT
{'person1': {'name': 'Rishabh', 'age': 26, 'city': 'nagpur'}, 'person2': {'name': 'Rohit', 'age': 25, 'city': 'nagpur'}}
print(nested_dict["person1"])
#OUTPUT
{'name': 'Rishabh', 'age': 26, 'city': 'nagpur'}
print(nested_dict["person1"]["name"])
#OUTPUT
Rishabh
Let’s learn about SET in PYTHON PROGRAMMING
A set is an unordered collection of unique elements. Sets are mutable, but unlike lists and dictionaries, they are unordered and do not support indexing.
Sets are commonly used for tasks such as removing duplicate elements from a list, performing set operations like union, intersection, difference, and symmetric difference, and testing membership efficiently
- Creation :- Sets are created by enclosing a comma-separated sequence of elements within curly braces { }
set = {1, 2, 3, 4, 5}
- Uniqueness :- Sets contain only unique elements. If you try to add an element that already exists in the set, it will not be added again.
my_set = {1, 2, 3, 4, 5, 1, 2}
print(my_set)
# Output
{1, 2, 3, 4, 5}
- Mutable :- Sets are mutable, meaning you can add or remove elements after the set has been created.
my_set={1,2,3,4,5}
my_set.add(6) # Add a single element
my_set.update([7, 8, 9]) # Add multiple elements
my_set.remove(3) # Remove an element
print(my_set)
#OUTPUT
{1, 2, 4, 5, 6, 7, 8, 9}
- Membership :- You can check if an element exists in a set using the in operator.
my_set={1,2,3,4,5}
print(2 in my_set)
#OUTPUT
True
- Length :- You can find the number of elements in a set using the len( ) function.
my_set={1,2,3,4,5}
print(len(my_set))
#OUTPUT
5
- Set Operations :- Sets support various set operations, including union (
|
), intersection (&
), difference (-
), and symmetric difference (^
).
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.union(set2)) OR print(set1|set2)
print(set1.intersection(set2)) OR print(set1&set2)
#OUTPUT.
{1, 2, 3, 4, 5}
{3}
Let’s learn about LOOPS in PYTHON PROGRAMMING
loops are used to execute a block of code repeatedly until a certain condition is met. There are two main types of loops in Python for loops and while loops.
- ‘for’ Loops :-
‘for’ loops are used to iterate over a sequence (such as a list, tuple, string, or dictionary) and execute a block of code for each item in the sequence.
#SYNTAX
for item in sequence:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
#OUTPUT
apple
banana
cherry
- ‘while’ Loops :-
‘while’ loops are used to execute a block of code repeatedly as long as a specified condition is true.
#SYNTAX
while condition:
while True :
print("rishabh")
#OUTPUT
it will print rishabh infinite times.
count=1
while count <=5 :
print("rishabh")
count+=1 #count increasing by 1
#OUTPUT
rishabh
rishabh
rishabh
rishabh
rishabh
iterator=1
while iterator <=5 :
print("rishabh",iterator)
iterator+=1 #count increasing by 1
#OUTPUT
rishabh 1
rishabh 2
rishabh 3
rishabh 4
rishabh 5
Lets solve some practice problems on while Loops.
- Print number from 1 to 100.
i=1
while i<=100 :
print(i)
i+=1
#OUTPUT
1
2
3
.
.
.
100
- Print number from 100 to 1.
i=100
while i>=1 : #stoping condition
print(i)
i-=1
#OUTPUT
100
99
97
.
.
.
1
- print the multiplication table of a number n.
i=1
while i<=10 :
print(3*i)
i+=1
#OUTPUT
3
6
9
12
15
18
21
24
27
30
- Print the elements of the following list using loop.
[1,4,9,16,25,36,49,64,81,100]
numb=[1,4,9,16,25,36,49,64,81,100]
i=0
while i<len(numb):
print(numb[i])
i+=1
#OUTPUT
1
4
9
16
25
36
49
64
81
100
Loop Control Statements:
‘break’ :- Terminates the loop prematurely.
‘continue’ :- Skips the rest of the code inside the loop for the current iteration and continues with the next iteration.
#break
i=1
while i<5:
print(i)
if(i==3):
break
i+=1
#OUTPUT
1
2
3
#continue
i=0
while i<=5:
if(i==3):
i+=1
continue #skip
print(i)
i+=1
#OUTPUT
0
1
2
4
5
- range( ) :- Range function written a sequence of numbers, starting from 0 by default, and increments by 1 (by default) and stop before specified number.
range(start?,stop,step?) #step size means by how much you want increase value
xyz=range(5) #ending number is not included
for i in xyz: OR for i in range(5):
print(i)
#OUTPUT
0
1
2
3
4
start? —> ? means optional, if we don’t want to give start value then it will take 0 by default.
stop —> It is compulsory to give stop value.
step? —> ? means optional, if we don’t want to give step value then it will take 1 by default.
for i in range(5): #range(stop)
for i in range(2,5): #range(start,stop)
for i in range(2,5,2): #range(start,stop,step)
- pass statement :-
pass is null statement that does nothing. It is used as a placeholder for future code.
Let’s learn about FUNCTIONS in PYTHON PROGRAMMING
A function is a block of reusable code that performs a specific task. Functions allow you to organize code into manageable pieces, promote code reuse, and make your code more modular and readable.
- Defining a Function :- You define a function using the def keyword followed by the function name and parentheses containing optional parameters. The function body is indented below the function definition.
def cal_sum(a,b):
sum=a+b
print(sum)
return sum
---------------------
#above code is entire function to calculate sum of a and b.
- Calling a Function :- To execute the code inside a function, you call the function by using its name followed by parentheses containing arguments (if any).
cal_sum(5,2) #this is a call function, the value given in the parentheses will store in a and b.
cal_sum(10,20)
cal_sum(5,7)
#OUTPUT
7
30
12
Same function can be used for multiple calculation, because of this redundancy will decrease.
#Another example.lets print hello.
def print_hello():
print(Hello)
print_hello() #calling function
#OUTPUT
Hello
There are two types of function one is built-in function eg. print( ), len( ) etc. User defined function which is created above.
Let’s learn about RECURSION in PYTHON PROGRAMMING
Recursion is a programming technique where a function calls itself in order to solve smaller instances of the same problem. It’s a powerful concept used in algorithms and programming languages like Python to solve problems that can be broken down into smaller, similar sub-problems. When a function calls itself repeatedly, it is similar to loop function.
#recursive function
def show(n):
if(n==0): #base case
return
print(n)
show(n-1)
show(5)
#OUTPUT
5
4
3
2
1
- Base case :- It prevents infinite recursion and provides a stopping condition for the recursion.
Let’s learn about I/O in PYTHON PROGRAMMING
- File I/O :- Python also supports reading from and writing to files using file objects and file-related functions like
open()
,read()
,write()
,close()
, etc. File I/O is commonly used for tasks like reading data from files, writing logs, and saving program state.
f=("file_name","mode")
#Example file_name=> sample.txt demo.docx etc mode=> r=read mode w=write mode.
if in case of mode forgot to mention then it will take as r mode by default.
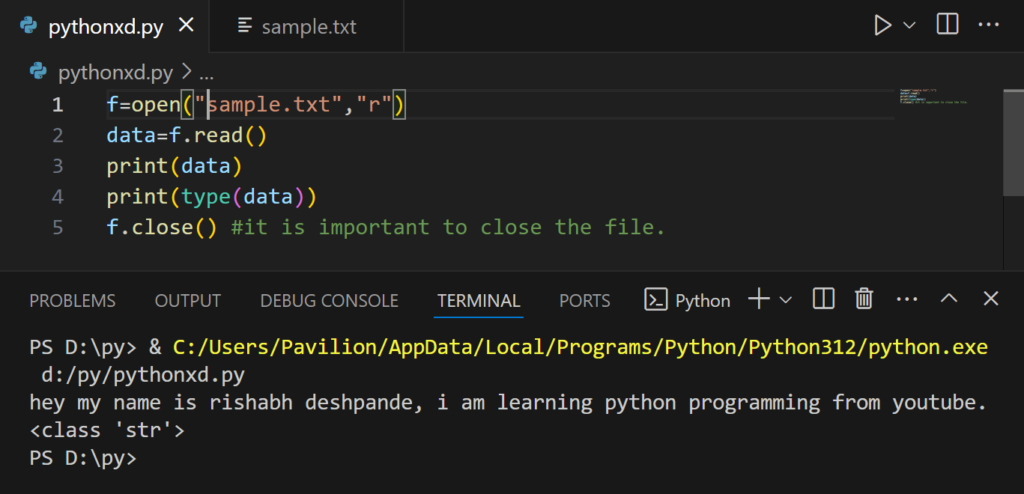
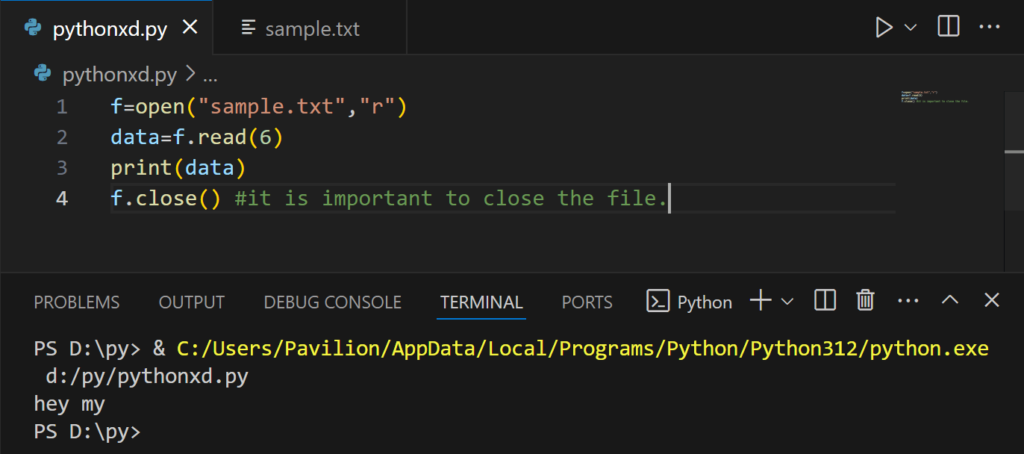
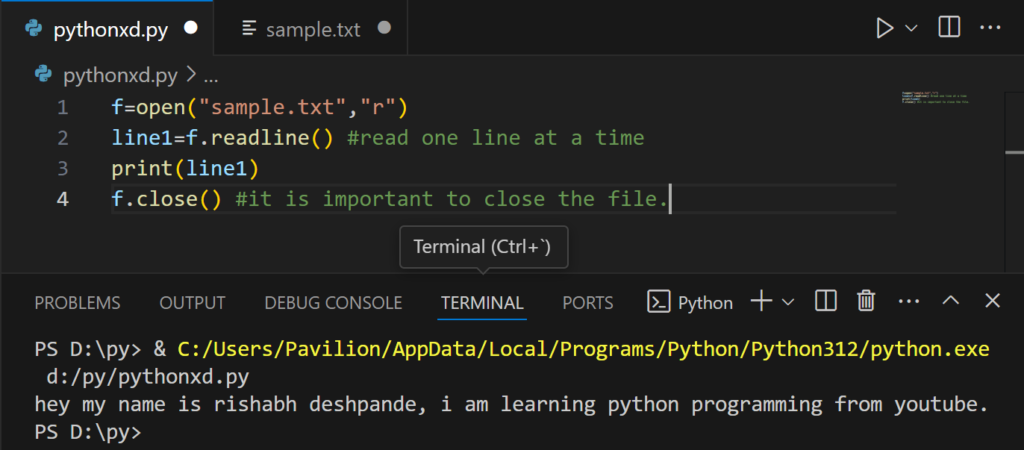
f=open("sample.txt","w")
f.write("hey how you doing") #overwrite the entire file.
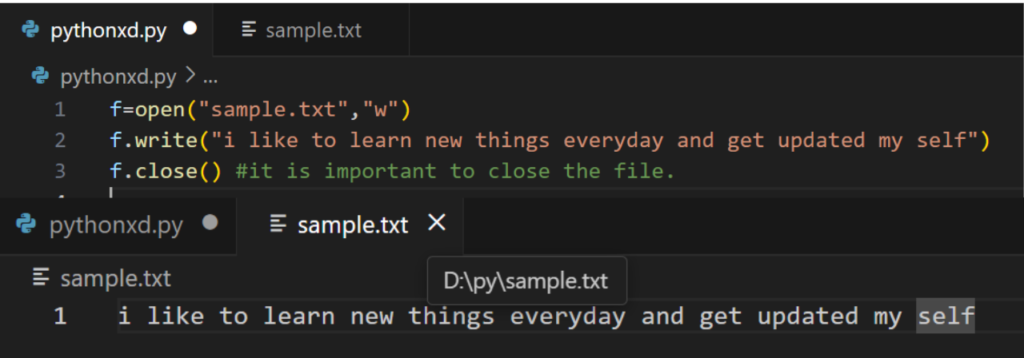
The argument mode points to a string beginning with one of the following sequences (Additional characters may follow these sequences.):
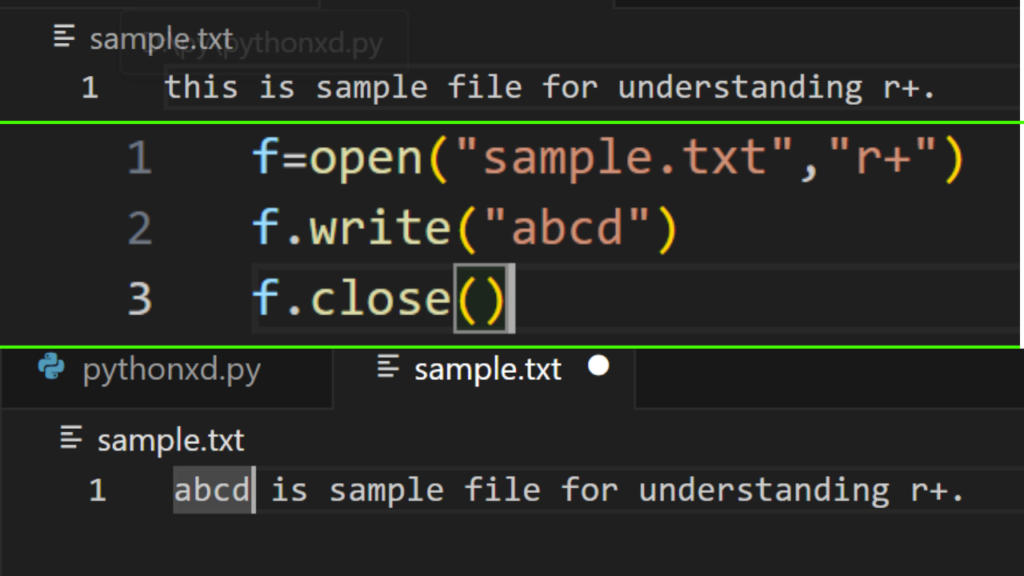
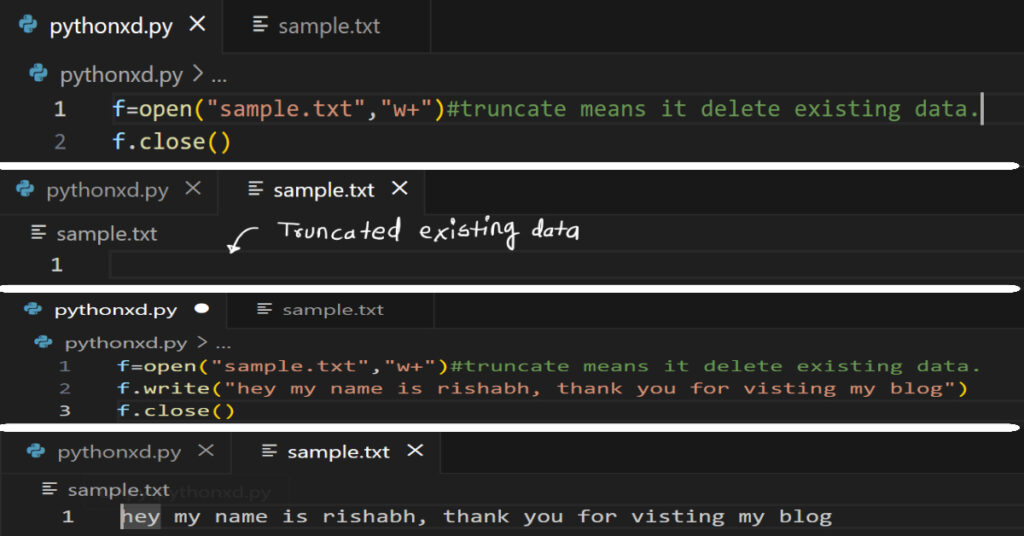